Voiced by Amazon Polly |
Joins are a crucial operation in any data processing pipeline, as they allow you to combine data from different datasets based on a common key. PySpark, the Python API for Apache Spark, is widely used for large-scale data processing and provides powerful join capabilities. However, joins can be computationally expensive, especially with large datasets, making optimization essential. In this blog, we’ll dive deep into how joins work in PySpark and explore various strategies to optimize them.
Customized Cloud Solutions to Drive your Business Success
- Cloud Migration
- Devops
- AIML & IoT
Understanding Joins in PySpark
PySpark supports several types of joins:
- Inner Join: Includes only matching rows from both datasets.
- Left Outer Join: Includes all rows from the left dataset and matching rows from the right dataset.
- Right Outer Join: Includes all rows from the right dataset and matching rows from the left dataset.
- Full Outer Join: Includes all rows from both datasets, with null in unmatched rows.
- Cross Join: Produces the Cartesian product of both datasets.
- Semi Join: Includes rows from the left dataset that match in the right dataset.
- Anti Join: Includes rows from the left dataset that do not match in the right dataset.
To perform a join in PySpark:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 |
from pyspark.sql import SparkSession # Initialize Spark Session spark = SparkSession.builder.appName("Join Optimization").getOrCreate() data1 = [(1, 'Alice', 1000), (2, 'Bob', 2000), (3, 'Cathy', 3000)] data2 = [(1, 'HR'), (2, 'Finance'), (4, 'Marketing')] schema1 = ["id", "name", "salary"] schema2 = ["id", "department"] df1 = spark.createDataFrame(data1, schema1) df2 = spark.createDataFrame(data2, schema2) # Perform an inner join joined_df = df1.join(df2, on="id", how="inner") joined_df.show() |
Challenges with Joins in PySpark
1. Data Skew:
Uneven distribution of keys can cause certain nodes to process significantly more data, leading to performance bottlenecks.
2. Shuffling:
Joins often involve shuffling data across nodes, which is a costly operation.
3. Memory Overhead:
Large datasets can exceed the available memory, leading to errors or degraded performance.
4. Join Type Selection:
Using an inappropriate join type can result in suboptimal performance.
Strategies for Optimizing Joins in PySpark
1. Broadcast Joins
For joins involving a small dataset and a large dataset, broadcasting the smaller dataset to all nodes can eliminate the need for shuffling:
from pyspark.sql.functions import broadcast
broadcasted_df2 = broadcast(df2)
joined_df = df1.join(broadcasted_df2, on=”id”, how=”inner”)
When to Use:
- The smaller dataset can fit in memory on each node.
- Significantly reduces shuffle and improves performance.
2. Salting Keys to Handle Skew
When keys in a dataset are unevenly distributed, adding a random salt to the keys can distribute the data more evenly:
from pyspark.sql.functions import lit, concat
salted_df1 = df1.withColumn(“salted_key”, concat(df1.id, lit(“_”), lit(“random”)))
salted_df2 = df2.withColumn(“salted_key”, concat(df2.id, lit(“_”), lit(“random”)))
joined_df = salted_df1.join(salted_df2, on=”salted_key”, how=”inner”)
3. Partitioning Data
Repartitioning the data based on the join key can optimize data locality and reduce shuffling:
partitioned_df1 = df1.repartition(“id”)
partitioned_df2 = df2.repartition(“id”)
joined_df = partitioned_df1.join(partitioned_df2, on=”id”, how=”inner”)
4. Avoiding Repeated Joins
Instead of performing multiple joins sequentially, combine the operations wherever possible to minimize shuffle stages:
combined_df = df1.join(df2, on=”id”, how=”inner”).join(df3, on=”id”, how=”inner”)
5. Using Optimized Data Formats
Use columnar storage formats like Parquet or ORC for faster data processing:
df1.write.format(“parquet”).save(“path/to/parquet”)
df2.write.format(“parquet”).save(“path/to/parquet”)
# Read and join
joined_df = spark.read.parquet(“path/to/parquet”).join(df2, on=”id”, how=”inner”)
6. Caching Intermediate Data
Cache intermediate results if they are reused multiple times:
df1.cache()
df2.cache()
joined_df = df1.join(df2, on=”id”, how=”inner”)
Monitoring and Debugging Joins
- Spark UI: Use the Spark UI to monitor stages, tasks, and shuffle operations.
- Explain Plan: Use .explain() to analyze the logical and physical plans of the join:
joined_df.explain(True)
Conclusion
Optimizing joins in PySpark is a combination of understanding your data, choosing the right join strategy, and leveraging Spark’s built-in capabilities effectively. By applying the techniques discussed in this blog, you can improve the performance and scalability of your PySpark pipelines. Remember to profile and monitor your jobs regularly to identify and address performance bottlenecks.
Get your new hires billable within 1-60 days. Experience our Capability Development Framework today.
- Cloud Training
- Customized Training
- Experiential Learning
About CloudThat
CloudThat is a leading provider of Cloud Training and Consulting services with a global presence in India, the USA, Asia, Europe, and Africa. Specializing in AWS, Microsoft Azure, GCP, VMware, Databricks, and more, the company serves mid-market and enterprise clients, offering comprehensive expertise in Cloud Migration, Data Platforms, DevOps, IoT, AI/ML, and more.
CloudThat is the first Indian Company to win the prestigious Microsoft Partner 2024 Award and is recognized as a top-tier partner with AWS and Microsoft, including the prestigious ‘Think Big’ partner award from AWS and the Microsoft Superstars FY 2023 award in Asia & India. Having trained 650k+ professionals in 500+ cloud certifications and completed 300+ consulting projects globally, CloudThat is an official AWS Advanced Consulting Partner, Microsoft Gold Partner, AWS Training Partner, AWS Migration Partner, AWS Data and Analytics Partner, AWS DevOps Competency Partner, AWS GenAI Competency Partner, Amazon QuickSight Service Delivery Partner, Amazon EKS Service Delivery Partner, AWS Microsoft Workload Partners, Amazon EC2 Service Delivery Partner, Amazon ECS Service Delivery Partner, AWS Glue Service Delivery Partner, Amazon Redshift Service Delivery Partner, AWS Control Tower Service Delivery Partner, AWS WAF Service Delivery Partner and many more.
To get started, go through our Consultancy page and Managed Services Package, CloudThat’s offerings.
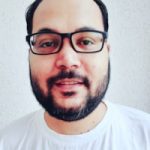
WRITTEN BY Pankaj Choudhary
Comments