Voiced by Amazon Polly |
Introduction
JavaScript, a dynamic and versatile programming language, is integral to the interactivity of modern web applications. To fully leverage its capabilities, it’s essential to understand its internal workings. This exploration delves into the JavaScript engine, the call stack, the event loop, and memory management.
Pioneers in Cloud Consulting & Migration Services
- Reduced infrastructural costs
- Accelerated application deployment
The JavaScript Engine
The JavaScript engine is at the heart of code execution, interpreting, and running JavaScript. Each browser has its own engine: Chrome uses V8, Firefox uses SpiderMonkey, Safari uses JavaScriptCore, and Microsoft Edge uses Chakra.
Parsing and Compilation
The execution process starts with parsing:
- Lexical Analysis (Tokenization): The source code is broken down into tokens, the smallest units such as keywords, operators, and identifiers.
- Syntax Analysis (Parsing): These tokens are parsed into an Abstract Syntax Tree (AST), which represents the hierarchical structure of the code.
Next comes compilation:
- Interpreter: Initially, an interpreter may quickly translate and execute the AST, but this can be slow for complex programs.
- Just-In-Time (JIT) Compilation: To optimize performance, modern engines use JIT compilation, which compiles code to machine language at runtime, optimizing based on actual usage patterns.
Optimization Techniques
JavaScript engines use various optimization methods:
- Hidden Classes and Inline Caching: These improve the performance of property access and method calls on objects.
- Garbage Collection: This process automatically reclaims memory no longer in use, preventing memory leaks.
The Call Stack
JavaScript operates as a single-threaded language, executing one command at a time, managed through the call stack:
- Call Stack: This data structure tracks function calls. When a function is called, it’s pushed onto the stack, and when it returns, it’s popped off.
Example
1 2 3 4 5 6 7 8 9 10 |
function first() { second(); console.log("First function"); } function second() { console.log("Second function"); } first(); |
Here’s the call stack in action:
- first() is called and pushed onto the stack.
- second() is called within first() and pushed onto the stack.
- second() executes, logs “Second function,” and is popped off.
- first() resumes, logs “First function,” and is popped off.
The Event Loop
Although JavaScript is single-threaded, it can handle asynchronous operations efficiently through the event loop. This allows for non-blocking operations, such as handling I/O, timers, and user interactions.
Components
- Web APIs: The browser provides these and handle asynchronous operations like setTimeout, HTTP requests, and DOM events.
- Callback Queue: Completed asynchronous operations place their callback functions in this queue.
- Event Loop: Continuously checks the call stack and callback queue. If the call stack is empty, the event loop moves the first callback from the queue to the stack for execution.
Example
1 2 3 4 5 6 7 |
console.log("Start"); setTimeout(() => { console.log("Timeout"); }, 1000); console.log("End"); |
Execution Flow:
- “Start” is logged.
- setTimeout registers a callback with a delay.
- “End” is logged.
- After the delay, the callback is moved to the call stack, and “Timeout” is logged.
Memory Management
JavaScript handles memory automatically through garbage collection, periodically freeing up memory used by objects no longer in use.
Garbage Collection Process
- Mark-and-Sweep Algorithm: This common method traverses the object graph, marking reachable objects and sweeping away unmarked ones.
- Reference Counting: Tracks the number of references to each object. When an object’s reference count drops to zero, it’s eligible for garbage collection.
1 2 3 4 5 6 |
let user = { name: "John" }; let admin = user; user = null; |
Initially, user references the object { name: “John” }. When user is set to null, the object is still referenced by admin, so it isn’t collected. If admin is also set to null, the object becomes unreachable and is collected.
Conclusion
This foundational knowledge aids developers in writing more efficient, optimized, and maintainable code.
Drop a query if you have any questions regarding JavaScript and we will get back to you quickly.
Empowering organizations to become ‘data driven’ enterprises with our Cloud experts.
- Reduced infrastructure costs
- Timely data-driven decisions
About CloudThat
CloudThat is a leading provider of Cloud Training and Consulting services with a global presence in India, the USA, Asia, Europe, and Africa. Specializing in AWS, Microsoft Azure, GCP, VMware, Databricks, and more, the company serves mid-market and enterprise clients, offering comprehensive expertise in Cloud Migration, Data Platforms, DevOps, IoT, AI/ML, and more.
CloudThat is recognized as a top-tier partner with AWS and Microsoft, including the prestigious ‘Think Big’ partner award from AWS and the Microsoft Superstars FY 2023 award in Asia & India. Having trained 650k+ professionals in 500+ cloud certifications and completed 300+ consulting projects globally, CloudThat is an official AWS Advanced Consulting Partner, Microsoft Gold Partner, AWS Training Partner, AWS Migration Partner, AWS Data and Analytics Partner, AWS DevOps Competency Partner, Amazon QuickSight Service Delivery Partner, Amazon EKS Service Delivery Partner, AWS Microsoft Workload Partners, Amazon EC2 Service Delivery Partner, and many more.
To get started, go through our Consultancy page and Managed Services Package, CloudThat’s offerings.
FAQs
1. What is the role of the JavaScript engine?
ANS: – The JavaScript engine is responsible for interpreting and executing JavaScript code. It includes parsing the code into an Abstract Syntax Tree (AST), compiling the code into machine language using Just-In-Time (JIT) compilation, and executing the compiled code.
2. How does JavaScript handle asynchronous operations if it is single-threaded?
ANS: – JavaScript uses the event loop to manage asynchronous operations. The event loop allows JavaScript to handle non-blocking operations by delegating tasks to Web APIs, placing callbacks in the callback queue, and executing these callbacks when the call stack is empty.
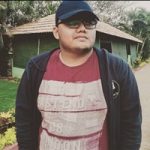
WRITTEN BY Subramanya Datta
Comments