Voiced by Amazon Polly |
Overview
AWS Lambda is the serverless computing service by Amazon Web Services, introduces Recursive Loop Detection APIs. This functionality helps developers manage and prevent infinite loops in their AWS Lambda functions, ensuring that resources are used efficiently and costs are controlled. This blog will overview the new APIs, discuss their purpose, prerequisites for use, implementation steps, and advantages, and conclude with some FAQs.
Pioneers in Cloud Consulting & Migration Services
- Reduced infrastructural costs
- Accelerated application deployment
Introduction
AWS Lambda is a service that allows you to run code without having to provision or manage servers because it is a serverless computing platform. However, one challenge with Lambda functions is correctly handling recursion, especially when working with services that trigger Lambda functions in a loop (such as Amazon SNS, Amazon SQS, or Amazon EventBridge). If not properly managed, recursive loops can lead to unintended high invocation rates, increased costs, and even service disruptions.
With the introduction of Recursive Loop Detection APIs, AWS Lambda now offers built-in mechanisms to detect and manage recursive invocation loops. These APIs provide developers with tools to identify potential recursions, set limits, and gracefully handle scenarios where recursion is detected.
Purpose of Recursive Loop Detection APIs
The primary purpose of the Recursive Loop Detection APIs is to prevent infinite loops within AWS Lambda functions that could cause unintended resource consumption and increased costs. The APIs are designed to:
- Detect Recursive Invocations: Identify when the AWS Lambda function is invoked recursively, directly or indirectly, and prevent it from running indefinitely.
- Set Recursive Limits: Allow developers to define maximum recursive invocation limits, providing greater control over function execution.
- Graceful Failure Handling: Offer mechanisms to handle scenarios when recursive limits are reached, such as sending notifications or triggering compensating workflows.
Prerequisites
Before using the Recursive Loop Detection APIs, ensure the following prerequisites are met:
- Need AWS Account: To create AWS Lambda functions, we need an active AWS account with the required permissions.
- AWS Lambda Function Set Up: The AWS Lambda function must be configured to handle the desired events.
- AWS IAM Role: A matching AWS IAM role that provides access to additional AWS services should, if applicable, be associated with the AWS Lambda function.
- AWS SDK: You need the latest version of the AWS SDK for your programming language to use the Recursive Loop Detection APIs.
Implementation Steps
Implementing Recursive Loop Detection APIs that include these steps:
- Identify Recursive Scenarios: Analyze your AWS Lambda function to identify potential recursion cases. For instance, mark these areas for recursive check implementation if your function processes events that might re-trigger the function.
- Enable Recursive Loop Detection: To set up loop detection for your AWS Lambda function, use the AWS SDK or AWS Management Console. This includes setting the maximum allowed recursive invocations. You can define these settings in the function’s configuration section.
1 2 3 4 5 6 7 8 9 10 11 12 |
import boto3 # Initialize the AWS SDK client for Lambda client = boto3.client('lambda') response = client.put_function_event_invoke_config( FunctionName='myLambdaFunction', MaximumRecursiveInvocations=10 # Set your limit ) print(response) |
- Handle Recursive Loop Detection in Code: Within your AWS Lambda function code, implement checks to handle cases where the recursion limit is reached. You might log a warning, send a notification, or trigger alternative logic.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
import json def lambda_handler(event, context): if 'RecursiveLimitExceeded' in event: print("Recursive limit exceeded, taking alternative action") # Implement alternative logic here return { 'statusCode': 429, 'body': json.dumps('Recursive limit exceeded') } # Normal function logic here print("Processing event") return { 'statusCode': 200, 'body': json.dumps('Success') } |
- Test Your Implementation: Deploy your AWS Lambda function and simulate scenarios where recursive invocation might occur. Ensure that your function correctly identifies and handles recursion.
Advantages
The introduction of Recursive Loop Detection APIs offers several advantages:
- Cost Control: By limiting recursive invocations, you can avoid unexpected costs associated with infinite loops.
- Resource Management: Ensures efficient use of resources by preventing unintended continuous execution of AWS Lambda functions.
- Improved Stability: Helps maintain the stability of your AWS environment by avoiding high invocation rates that could disrupt other services.
- Ease of Management: The APIs provide built-in support for recursion detection, reducing the need for custom implementation and monitoring logic.
Conclusion
By preventing infinite loops and offering mechanisms for handling recursive invocations, these APIs help maintain the stability, performance, and cost-effectiveness of serverless architectures.
Drop a query if you have any questions regarding AWS Lambda and we will get back to you quickly.
Experience Effortless Cloud Migration with Our Expert Solutions
- Stronger security
- Accessible backup
- Reduced expenses
About CloudThat
CloudThat is a leading provider of Cloud Training and Consulting services with a global presence in India, the USA, Asia, Europe, and Africa. Specializing in AWS, Microsoft Azure, GCP, VMware, Databricks, and more, the company serves mid-market and enterprise clients, offering comprehensive expertise in Cloud Migration, Data Platforms, DevOps, IoT, AI/ML, and more.
CloudThat is the first Indian Company to win the prestigious Microsoft Partner 2024 Award and is recognized as a top-tier partner with AWS and Microsoft, including the prestigious ‘Think Big’ partner award from AWS and the Microsoft Superstars FY 2023 award in Asia & India. Having trained 650k+ professionals in 500+ cloud certifications and completed 300+ consulting projects globally, CloudThat is an official AWS Advanced Consulting Partner, Microsoft Gold Partner, AWS Training Partner, AWS Migration Partner, AWS Data and Analytics Partner, AWS DevOps Competency Partner, AWS GenAI Competency Partner, Amazon QuickSight Service Delivery Partner, Amazon EKS Service Delivery Partner, AWS Microsoft Workload Partners, Amazon EC2 Service Delivery Partner, Amazon ECS Service Delivery Partner, AWS Glue Service Delivery Partner, Amazon Redshift Service Delivery Partner, AWS Control Tower Service Delivery Partner, AWS WAF Service Delivery Partner and many more.
To get started, go through our Consultancy page and Managed Services Package, CloudThat’s offerings.
FAQs
1. What happens if the recursion limit is reached?
ANS: – If the recursive limit is reached, AWS Lambda can trigger predefined actions such as logging an error, sending a notification, or executing compensating workflows. You can include custom logic in your function to deal with this situation.
2. Can I change the recursion limit dynamically?
ANS: – Yes, the recursion limit can be updated dynamically using the AWS SDK or through the AWS Management Console.
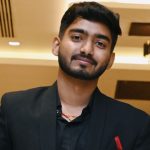
WRITTEN BY Rohit Kumar
Rohit Kumar works as a Research Associate (Infra, Migration, and Security Team) at CloudThat. He is focused on gaining knowledge of the Cloud environment. He has a keen interest in learning and researching emerging technologies.
Comments