Voiced by Amazon Polly |
Introduction
Microservices are a popular architectural style for building scalable, Reliable, and resilient applications. However, microservices have some issues, such as how to coordinate and communicate between different microservices. One of the popular ways to address this challenge is to use asynchronous communication between different microservices, which means that the sender and the receiver of a message do not have to wait for each other for their execution.
Your Cloud Experience with CloudThat’s Cloud Native Development Expertise
- Scalability
- Agility
- Optimize Performance
What is Asynchronous Communication?
Asynchronous communication is when the sender and the receiver of a message do not have to wait for each other for their execution. This communication allows microservices to interact without waiting for immediate responses, making the system more efficient. Asynchronous communication between microservices ensures that services are decoupled, resilience, high availability, and failures are handled.
How Asynchronous Communication Happens in Spring Boot
Asynchronous communication can be implemented between microservices in Spring Boot, focusing on HTTP-based non-blocking communication using WebClient and @Async methods, without relying on message brokers like Kafka, RabbitMQ, or ActiveMQ.
Asynchronous communication means that the sender doesn’t need to wait for the recipient to process the request and respond. Instead, the sender can continue its processing, and when the response is available, it will handle it accordingly. This pattern is common in microservice architectures where services need to perform tasks in parallel.
In Spring Boot, asynchronous communication can be implemented using:
- WebClient: A non-blocking HTTP client that enables reactive programming.
- @Async: A Spring annotation that runs methods asynchronously in a separate thread.
These tools play a critical role in implementing non-blocking, reactive, and asynchronous communication patterns within distributed systems.
WebClient
WebClient is part of Spring WebFlux and is designed to make asynchronous and non-blocking HTTP requests. It replaces the traditional RestTemplate, which is synchronous and blocking. WebClient provides more flexibility and is reactive by design, making it ideal for handling a large number of concurrent requests with minimal resource consumption.
Key Features of WebClient:
- Non-blocking I/O: It doesn’t tie up threads while waiting for the response.
- Reactive programming: Supports reactive streams and integrates seamlessly with Mono and Flux
- Customization: Supports filters, interceptors, and custom serializers.
- Error handling: Provides a more granular way to handle errors at different stages.
Async: Asynchronous Method Execution
- The @Async annotation in Spring is used to run a method asynchronously, meaning that the calling thread will not block while the annotated method runs in the background. Instead, the method executes in a different thread provided by a Spring-managed task executor.
Key Features of WebClient
Non-Blocking I/O:
• WebClient operates in a non-blocking manner. This means that when a request is made to an external service, the thread making the call is not blocked and is waiting for the response. Instead, the request is executed asynchronously, and the thread is free to process other tasks.
• How it works: When an HTTP request is made, the client subscribes to a publisher that eventually produces the response. Once the response is ready, the client is notified asynchronously, and it can continue processing the result.
Reactive Streams Support (Project Reactor Integration)
• WebClient is built on top of Project Reactor, a reactive programming library that implements the Reactive Streams specification. This allows you to work with Mono and Flux types for handling single or multiple asynchronous results.
Mono: Represents a single value or no value (similar to an Optional).
Flux: Represents a stream of 0 or more values.
• This makes WebClient a natural fit for modern applications that require responsiveness and scalability when dealing with streams of data or working with high-concurrency systems.
Customization
• It allows deep customization of headers, query parameters, request bodies, and even serialization mechanisms. You can set default headers, attach query parameters, configure timeouts, and define custom serialization and deserialization mechanisms.
Built-in Error Handling
• Handling errors (such as HTTP 4xx and 5xx responses) is crucial in microservices and distributed applications. It provides a clean API to handle such errors at different stages in the request pipeline. As a developer, I can define custom logic for handling specific status codes and exceptions.
Filters and Interceptors
• It supports filters that can be used to intercept requests and responses, which is useful for cross-cutting concerns like logging, monitoring, authentication, and retry mechanisms. Filters are similar to interceptors in traditional web applications.
Key Features of @Async
Asynchronous Method Execution
• When a method is annotated with @Async, it runs in a separate thread, freeing up the main thread to continue executing other tasks.
Concurrency Control with Thread Pool
• Spring uses a thread pool to execute the tasks asynchronously. By default, Spring provides a simple thread pool, but you can customize it by defining your own Executor. This allows you to control the number of concurrent tasks that can be executed and tune the pool size according to the application’s load requirements
Non-Blocking Operations
• @Async allows non-blocking operations, meaning that the calling thread is not held up waiting for the result of the asynchronous method. This enhances application responsiveness and reduces latency, particularly for I/O-heavy operations like HTTP requests or database interactions.
Supports Multiple Return Types
The @Async method can return several types, allowing for flexible usage depending on the scenario:
• void: The method runs asynchronously, and the result is not needed.
• Future: This provides a handle to the result, which can be retrieved later by calling future.get(). It blocks until the result is available.
• CompletableFuture: A more advanced option, it supports non-blocking ways to handle the result when it’s ready. It also allows tasks to be chained together asynchronously using methods like thenApply(), thenAccept(), and exceptionally().
• ListenableFuture: Similar to CompletableFuture, this is provided by Spring to handle results asynchronously.
Error Handling with Exception Propagation
• Exceptions thrown in asynchronous methods are not propagated to the caller. However, you can handle errors using CompletableFuture.exceptionally() or by defining an AsyncUncaughtExceptionHandler to catch and manage exceptions in asynchronous methods.
Conclusion
Asynchronous communication plays a pivotal role in ensuring that microservices are decoupled, resilient, and highly available. It enables services to communicate without blocking or waiting for responses, leading to improved system efficiency and performance. When applied correctly, asynchronous communication allows for
- Increased resilience: Services are no longer dependent on each other for immediate responses, reducing the risk of cascading failures.
- Better fault tolerance: Asynchronous systems are better equipped to handle service outages, network issues, and other failures without causing bottlenecks.
- Scalability: Since threads are not blocked waiting for responses, the system can handle a large number of concurrent requests, making it more scalable.
Attend 8+ DevOps and Kubernetes Certification Trainings and become a Certified DevOps expert
- Experienced Authorized Instructor led Training
- Live Hands-on Labs
About CloudThat
CloudThat is a leading provider of Cloud Training and Consulting services with a global presence in India, the USA, Asia, Europe, and Africa. Specializing in AWS, Microsoft Azure, GCP, VMware, Databricks, and more, the company serves mid-market and enterprise clients, offering comprehensive expertise in Cloud Migration, Data Platforms, DevOps, IoT, AI/ML, and more.
CloudThat is the first Indian Company to win the prestigious Microsoft Partner 2024 Award and is recognized as a top-tier partner with AWS and Microsoft, including the prestigious ‘Think Big’ partner award from AWS and the Microsoft Superstars FY 2023 award in Asia & India. Having trained 650k+ professionals in 500+ cloud certifications and completed 300+ consulting projects globally, CloudThat is an official AWS Advanced Consulting Partner, Microsoft Gold Partner,AWS Training Partner,AWS Migration Partner,AWS Data and Analytics Partner,AWS DevOps Competency Partner,AWS GenAI Competency Partner,Amazon QuickSight Service Delivery Partner,Amazon EKS Service Delivery Partner, AWS Microsoft Workload Partners,Amazon EC2 Service Delivery Partner,Amazon ECS Service Delivery Partner,AWS Glue Service Delivery Partner,Amazon Redshift Service Delivery Partner,AWS Control Tower Service Delivery Partner,AWS WAF Service Delivery Partner and many more.
To get started, go through our Consultancy page and Managed Services Package,CloudThat’s offerings.
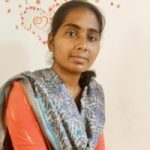
WRITTEN BY P Santhiya
Comments