Voiced by Amazon Polly |
Overview
Python is one of the most popular programming languages in the world today, known for its simplicity, versatility, and readability. However, one often overlooked limitation of Python is the Global Interpreter Lock (GIL). The GIL is a mutex that allows only one thread to execute Python bytecode at a time, even on multi-core systems. While it helps maintain the integrity of Python’s memory management, it can also be a significant bottleneck for multi-threaded applications that must take full advantage of modern hardware. This blog explores the problem of the GIL and discusses various strategies to overcome it, enabling developers to boost the performance of their Python programs.
Pioneers in Cloud Consulting & Migration Services
- Reduced infrastructural costs
- Accelerated application deployment
Understanding the Global Interpreter Lock (GIL)
Before we dive into overcoming the GIL, it’s crucial to understand what it is and why it exists.
The GIL is a mechanism used by CPython, the reference implementation of Python, to protect access to Python objects, ensuring that only one thread executes Python bytecode at any given time. This lock simplifies the implementation of CPython by preventing race conditions that might occur in multi-threaded programs. While the GIL ensures thread safety, it also limits the performance of CPU-bound multi-threaded programs. On a multi-core machine, only one CPU core is utilized effectively when running Python code, even with multiple threads.
For I/O-bound tasks, such as reading from a file or making network requests, the GIL is less of an issue because threads often spend much time waiting on I/O operations. However, the GIL can become a major hindrance for CPU-bound tasks like complex calculations or data processing.
Why the GIL Matters?
The GIL presents a significant challenge for developers building multi-threaded Python applications. Here are some key reasons why the GIL can negatively impact performance:
- Single Threaded Execution: Only one thread can execute Python bytecode at a time, meaning that multi-threading doesn’t improve performance for CPU-bound tasks.
- Underutilization of Multi-core CPUs: Modern processors come with multiple cores, but the GIL prevents Python from fully utilizing all the cores. This is particularly problematic for applications that require parallel processing.
- Concurrency Issues: When dealing with multi-threaded code, even though threads may be concurrently waiting for resources (such as I/O operations), only one thread can be running at any point, leading to inefficiencies.
Overcoming the GIL: Strategies and Techniques
While the GIL can hinder multi-threaded performance, several techniques can be employed to work around it and maximize the performance of Python applications.
- Multiprocessing
One of the most effective ways to bypass the GIL is to use Python’s multiprocessing module, which allows you to create multiple processes, each with its own Python interpreter and memory space. Since the GIL is specific to each process, multiple processes can run in parallel on multiple CPU cores, making it a great solution for CPU-bound tasks.
With multiprocessing, you can use multi-core systems and run Python code concurrently in separate processes. Here’s an example:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
import multiprocessing def worker(num): print(f'Worker {num} is processing') if __name__ == '__main__': processes = [] for i in range(4): process = multiprocessing.Process(target=worker, args=(i,)) processes.append(process) process.start() for process in processes: process.join() |
In this example, four processes are created, each running concurrently. This method sidesteps the GIL by using separate memory spaces for each process.
- Cython and Native Extensions
Cython is a superset of Python that allows you to write Python code that is compiled into C. It can be used to optimize CPU-bound code by allowing you to write C extensions for performance-critical parts of your code. Since Cython allows you to release the GIL for certain operations, you can achieve true parallelism by leveraging native C code while still working within the Python ecosystem.
For example, you can write a Cython function that performs intensive calculations and releases the GIL during the computation to allow other threads or processes to run simultaneously.
Here’s a simple example of a Cython function:
1 2 3 4 5 6 |
# mymodule.pyx def compute(): cdef int i for i in range(1000000): # perform heavy computation pass |
You can compile this Cython code into a shared object and call it from Python, gaining a performance boost while reducing the impact of the GIL.
- Threading with I/O-bound Tasks
As mentioned, the GIL doesn’t significantly impact I/O-bound tasks, such as network requests or file operations. If your application is I/O-heavy, using the threading module can still be effective, as threads spend much more time waiting for external resources than running Python bytecode.
For example, when you make HTTP requests or perform file operations in a multi-threaded environment, you can see performance gains by creating multiple concurrent threads.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
import threading import requests def fetch_data(url): response = requests.get(url) print(f'Data fetched from {url}') if __name__ == '__main__': urls = ['http://example.com', 'http://example.org'] threads = [] for url in urls: thread = threading.Thread(target=fetch_data, args=(url,)) threads.append(thread) thread.start() for thread in threads: thread.join() |
In this example, threads are used to fetch data from multiple URLs concurrently, but since the tasks are I/O-bound, the GIL isn’t a limiting factor.
- Asyncio
For handling high-level I/O-bound tasks with concurrency, Python’s asyncio module is a powerful tool. asyncio allows you to write asynchronous code using the async/await syntax, providing a non-blocking event loop for managing I/O operations. This is particularly useful for handling thousands of simultaneous network connections or performing non-blocking I/O operations.
Unlike threading, asyncio doesn’t require multiple threads or processes. Instead, it runs within a single thread, avoiding thread management overhead and still achieving high concurrency. Since the GIL isn’t blocking during I/O operations, asyncio can make Python applications much more scalable.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
import asyncio async def fetch_data(url): print(f'Fetching {url}') await asyncio.sleep(1) # Simulate I/O print(f'Data fetched from {url}') async def main(): urls = ['http://example.com', 'http://example.org'] tasks = [fetch_data(url) for url in urls] await asyncio.gather(*tasks) if __name__ == '__main__': asyncio.run(main()) |
This code concurrently fetches data from multiple URLs using asynchronous tasks, making it efficient without requiring multiple threads.
- Using Other Python Implementations
While CPython (the default Python implementation) uses the GIL, other Python implementations, such as Jython (Python on the JVM) and IronPython (Python on .NET), do not have a GIL. If the performance bottleneck results from the GIL and multi-threading is critical to your application, you could consider using an alternative Python implementation. However, compatibility with third-party libraries may be a concern in such cases.
Conclusion
However, several strategies are available for overcoming the GIL, such as using multiprocessing, Cython, threading for I/O-bound tasks, asyncio, or considering alternative Python implementations. By choosing the right approach based on the nature of your task, you can significantly improve the performance of Python applications and better use modern multi-core processors.
Drop a query if you have any questions regarding Global Interpreter Lock and we will get back to you quickly.
Empowering organizations to become ‘data driven’ enterprises with our Cloud experts.
- Reduced infrastructure costs
- Timely data-driven decisions
About CloudThat
CloudThat is a leading provider of Cloud Training and Consulting services with a global presence in India, the USA, Asia, Europe, and Africa. Specializing in AWS, Microsoft Azure, GCP, VMware, Databricks, and more, the company serves mid-market and enterprise clients, offering comprehensive expertise in Cloud Migration, Data Platforms, DevOps, IoT, AI/ML, and more.
CloudThat is the first Indian Company to win the prestigious Microsoft Partner 2024 Award and is recognized as a top-tier partner with AWS and Microsoft, including the prestigious ‘Think Big’ partner award from AWS and the Microsoft Superstars FY 2023 award in Asia & India. Having trained 650k+ professionals in 500+ cloud certifications and completed 300+ consulting projects globally, CloudThat is an official AWS Advanced Consulting Partner, Microsoft Gold Partner, AWS Training Partner, AWS Migration Partner, AWS Data and Analytics Partner, AWS DevOps Competency Partner, AWS GenAI Competency Partner, Amazon QuickSight Service Delivery Partner, Amazon EKS Service Delivery Partner, AWS Microsoft Workload Partners, Amazon EC2 Service Delivery Partner, Amazon ECS Service Delivery Partner, AWS Glue Service Delivery Partner, Amazon Redshift Service Delivery Partner, AWS Control Tower Service Delivery Partner, AWS WAF Service Delivery Partner, Amazon CloudFront, Amazon OpenSearch, AWS DMS, AWS Systems Manager, Amazon RDS, and many more.
FAQs
1. How can one overcome the GIL for CPU-bound tasks in Python?
ANS: – One of the most effective solutions to overcome the GIL for CPU-bound tasks is to use the multiprocessing module. This allows you to create multiple processes, each with its own Python interpreter and memory space, bypassing the GIL and enabling true parallel execution on multi-core systems. Each process runs independently, so they can fully utilize multiple CPU cores without being hindered by the GIL.
2. Can one use Python's concurrent.futures module to bypass the GIL?
ANS: – Yes, you can use the concurrent.futures module to overcome the GIL for parallel execution, but it depends on the task. For CPU-bound tasks, you should use the ProcessPoolExecutor from the concurrent.futures module, which uses separate processes rather than threads. This avoids the GIL and allows you to use multiple CPU cores. For I/O-bound tasks, the ThreadPoolExecutor can still work well, as the GIL is released during I/O operations, allowing threads to run concurrently.
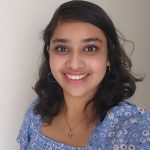
WRITTEN BY Hridya Hari
Hridya Hari works as a Research Associate - Data and AIoT at CloudThat. She is a data science aspirant who is also passionate about cloud technologies. Her expertise also includes Exploratory Data Analysis.
Comments